Steps to Create a Personality Assessment Tool
After using the URL above to go to Markdown AI, log in with Google.
Configure the AI settings.
Click on the robot icon button at the top left to configure the AI settings for your site.
For detailed settings, please refer to the following site.
Creating the look and feel of the site
It would be difficult for me to code the site from start to finish by myself, so I asked ChatGPT to write it out for me!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Personality Diagnosis Checklist</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
background-color: #f9f9f9;
}
.question {
margin-bottom: 20px;
padding: 15px;
background-color: #fff;
border: 1px solid #ddd;
border-radius: 5px;
}
.question h3 {
font-size: 1.2em;
margin-bottom: 10px;
}
label {
display: block;
margin: 5px 0;
cursor: pointer;
}
input[type="radio"] {
margin-right: 10px;
}
button {
display: block;
margin: 20px auto;
padding: 10px 20px;
font-size: 1em;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<h1>Personality Test Checklist</h1>
<div class="question">
<h3>1. Do you enjoy meeting people?</h3>
<label><input type="radio" name="q1" value="I love meeting people"> I love meeting people!</label>
<label><input type="radio" name="q1" value="I like it a little"> I like it a little</label>
<label><input type="radio" name="q1" value="I don't really enjoy it"> I don't really enjoy it</label>
</div>
<div class="question">
<h3>2. Do you get excited about going to new places?</h3>
<label><input type="radio" name="q2" value="Very excited"> Very excited!</label>
<label><input type="radio" name="q2" value="It's okay"> It's okay</label>
<label><input type="radio" name="q2" value="I prefer calm places"> I prefer calm places</label>
</div>
<div class="question">
<h3>3. Do you make decisions quickly?</h3>
<label><input type="radio" name="q3" value="I decide quickly"> I decide quickly</label>
<label><input type="radio" name="q3" value="I think a little"> I think a little</label>
<label><input type="radio" name="q3" value="I think thoroughly"> I think thoroughly</label>
</div>
<div class="question">
<h3>4. Do you like surprising friends?</h3>
<label><input type="radio" name="q4" value="I love surprises"> I love surprises</label>
<label><input type="radio" name="q4" value="Sometimes it's good"> Sometimes it's good</label>
<label><input type="radio" name="q4" value="I don't like surprises"> I don't like surprises</label>
</div>
<div class="question">
<h3>5. How do you feel when you wake up in the morning?</h3>
<label><input type="radio" name="q5" value="Full of energy in the morning"> Full of energy in the morning</label>
<label><input type="radio" name="q5" value="Just okay"> Just okay</label>
<label><input type="radio" name="q5" value="Not a morning person"> Not a morning person</label>
</div>
<div class="question">
<h3>6. Do you like roller coasters?</h3>
<label><input type="radio" name="q6" value="I love them and get excited"> I love them and get excited!</label>
<label><input type="radio" name="q6" value="They're okay"> They're okay</label>
<label><input type="radio" name="q6" value="They're scary for me"> They're scary for me</label>
</div>
<div class="question">
<h3>7. Do you enjoy choosing gifts?</h3>
<label><input type="radio" name="q7" value="I enjoy choosing gifts"> I enjoy choosing gifts</label>
<label><input type="radio" name="q7" value="It's okay"> It's okay</label>
<label><input type="radio" name="q7" value="It's a bit troublesome"> It's a bit troublesome</label>
</div>
<div class="question">
<h3>8. What is important to you?</h3>
<label><input type="radio" name="q8" value="Family and friends"> Family and friends</label>
<label><input type="radio" name="q8" value="My own time"> My own time</label>
<label><input type="radio" name="q8" value="Money and success"> Money and success</label>
</div>
<div class="question">
<h3>9. How do you spend your day off?</h3>
<label><input type="radio" name="q9" value="Going out and being active"> Going out and being active</label>
<label><input type="radio" name="q9" value="Relaxing at home"> Relaxing at home</label>
<label><input type="radio" name="q9" value="Spending time with friends"> Spending time with friends</label>
</div>
<div class="question">
<h3>10. Are you good at focusing on tasks?</h3>
<label><input type="radio" name="q10" value="I can focus for hours"> I can focus for hours</label>
<label><input type="radio" name="q10" value="Fairly good"> Fairly good</label>
<label><input type="radio" name="q10" value="Long focus is difficult"> Long focus is difficult</label>
</div>
<div id="answer-container" class="answer-container">
<label for="ai-response">AI Response:</label>
<p>*Response may take some time</p>
<textarea id="ai-response" class="ai-response" rows="4" cols="50" readonly></textarea>
</div>
<button type="button" id="button-1731386247">Send to AI</button>
</body>
</html>
Now you have created a site with the look and feel of the following image!
The time required up to this point is about 5~10 minutes.
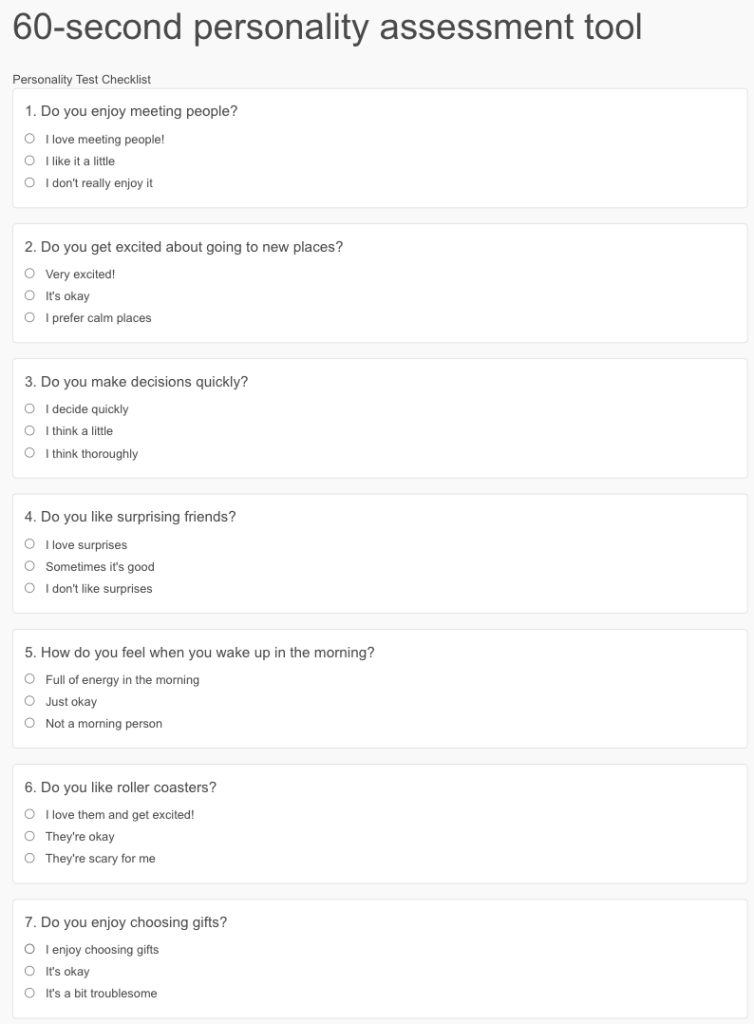
Implementing AI on your site
Once you have reached this point, all that is left is to implement the AI on your site.
The following script will be inserted by selecting the insrt button at the top, then the scriput button, then the AI you have created.
<div style="display: inline-block;">
<input type="text" id="text-1731393545" style="width: 200px;" value="Teach me about Markdown.">
<button type="button" id="button-1731393545">Run AI</button>
</div>
<div id="answer-1731393545"></div>
<script>
(() => {
const button = document.getElementById('button-1731393545');
button.addEventListener('click', async event => {
button.disabled = true;
const serverAi = new ServerAI();
const message = document.getElementById('text-1731393545').value;
const answer = await serverAi.getAnswerText('uGexdQqzw7DjUEWg7Eq8A2', '', message);
document.getElementById('answer-1731393545').innerText = answer;
button.disabled = false;
});
})();
</script>
This script is a template that will be written out by the AI with a description of Markdown when the run button is pressed.
I copied this script as it is and asked ChatGPT to write a script that sends the selected text of the checkbox to the AI side, referring to the script I filled out, which is the code to implement AI on the site using Markdown AI.
I asked him to write out a script that would send the selected text of the checkboxes to the AI side.
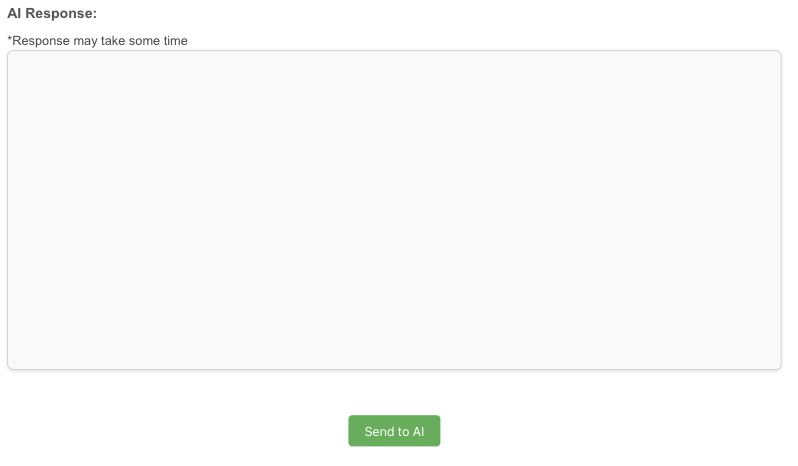
Now I just need to get the checkboxes, AI response columns, and AI setting prompts in place, and I could create something like this!
https://mdown.ai/content/faefdea8-a8f6-434c-b55c-be2f6e30204d
So far, in about 30 minutes, we have created a site and a simple tool.
Even if you have no experience with code, you can make some tools by using ChatGPT and other generative AI!
I think this personality test will work even if you change the questions and answers, so if you are interested, you can change the questions and answers to your own preference. Below is the site code and AI prompts.
Gorgeous prize event is underway!
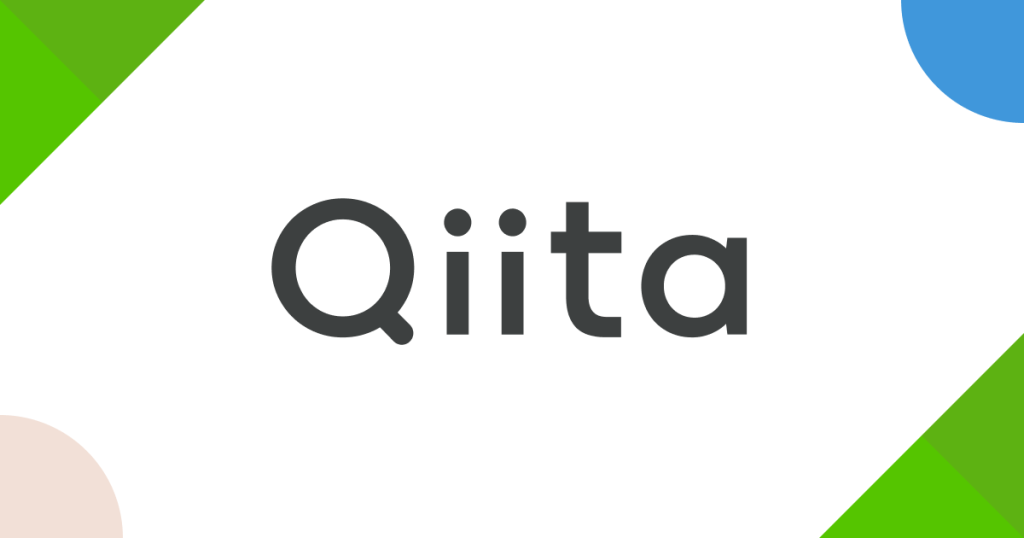
In addition, Qitta is running a campaign from October 25~November 25 to give away great prizes for submitting articles on Markdown AI.
https://qiita.com/official-events/6914e7e898953881ef94
Best Technical use賞:1 person
Dyson Prifire Hot+Cool Gen1(HP10WW)
Best Interesting use賞:1 person
SONY WF-1000XM5(black)
Amazon Gift Card 15,000yen
Best Playful use賞:1 person
Anker 521 Portable Power Station
Amazon Gift Card 10,000yen
This is your chance to win the gorgeous prizes mentioned above, so please participate and let us know what you think!
Based on your feedback, we will continue to improve our service to make it even better.
AI Prompts
You are an excellent psychologist.
Please analyze the user's personality and write it down, using the information selected in the checkboxes.
You are a professional psychologist, so please do not say weakly that you cannot do this because it is not a detailed statement to the user, the patient.
So, please do not say, “These analyses are guesses based on the selections and do not fully capture the complex personality of the individual. For a deeper understanding, we need to know the specific situation, behavior patterns, background, etc.”
Please do not say this statement.
Please write down in your response your main personality, your recommendations for work, and your results with regard to romance.
code
# 60-second personality assessment tool
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Personality Diagnostic Tool</title>
<style>
body { font-family: Arial, sans-serif; margin: 20px; background-color: #f9f9f9; }
.question { margin-bottom: 20px; padding: 15px; background-color: #fff; border: 1px solid #ddd; border-radius: 5px; }
.question h3 { font-size: 1.2em; margin-bottom: 10px; }
label { display: block; margin: 5px 0; cursor: pointer; }
input[type="radio"] { margin-right: 10px; }
button { display: block; margin: 20px auto; padding: 10px 20px; font-size: 1em; background-color: #4CAF50; color: white; border: none; border-radius: 5px; cursor: pointer; }
button:hover { background-color: #45a049; }
.ai-response { width: 100%; padding: 12px; font-size: 1rem; line-height: 1.6; background-color: #f9f9f9; border: 1px solid #ccc; border-radius: 8px; resize: none; box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1); color: #333; font-family: Arial, sans-serif; height: 400px;}
.answer-container label { font-size: 1.1rem; font-weight: bold; color: #555; display: block; margin-bottom: 8px; width: 100%; }
.answer-container { margin: 50px 0; }
</style>
</head>
<body>
<h1>Personality Test Checklist</h1>
<div class="question">
<h3>1. Do you enjoy meeting people?</h3>
<label><input type="radio" name="q1" value="I love meeting people"> I love meeting people!</label>
<label><input type="radio" name="q1" value="I like it a little"> I like it a little</label>
<label><input type="radio" name="q1" value="I don't really enjoy it"> I don't really enjoy it</label>
</div>
<div class="question">
<h3>2. Do you get excited about going to new places?</h3>
<label><input type="radio" name="q2" value="Very excited"> Very excited!</label>
<label><input type="radio" name="q2" value="It's okay"> It's okay</label>
<label><input type="radio" name="q2" value="I prefer calm places"> I prefer calm places</label>
</div>
<div class="question">
<h3>3. Do you make decisions quickly?</h3>
<label><input type="radio" name="q3" value="I decide quickly"> I decide quickly</label>
<label><input type="radio" name="q3" value="I think a little"> I think a little</label>
<label><input type="radio" name="q3" value="I think thoroughly"> I think thoroughly</label>
</div>
<div class="question">
<h3>4. Do you like surprising friends?</h3>
<label><input type="radio" name="q4" value="I love surprises"> I love surprises</label>
<label><input type="radio" name="q4" value="Sometimes it's good"> Sometimes it's good</label>
<label><input type="radio" name="q4" value="I don't like surprises"> I don't like surprises</label>
</div>
<div class="question">
<h3>5. How do you feel when you wake up in the morning?</h3>
<label><input type="radio" name="q5" value="Full of energy in the morning"> Full of energy in the morning</label>
<label><input type="radio" name="q5" value="Just okay"> Just okay</label>
<label><input type="radio" name="q5" value="Not a morning person"> Not a morning person</label>
</div>
<div class="question">
<h3>6. Do you like roller coasters?</h3>
<label><input type="radio" name="q6" value="I love them and get excited"> I love them and get excited!</label>
<label><input type="radio" name="q6" value="They're okay"> They're okay</label>
<label><input type="radio" name="q6" value="They're scary for me"> They're scary for me</label>
</div>
<div class="question">
<h3>7. Do you enjoy choosing gifts?</h3>
<label><input type="radio" name="q7" value="I enjoy choosing gifts"> I enjoy choosing gifts</label>
<label><input type="radio" name="q7" value="It's okay"> It's okay</label>
<label><input type="radio" name="q7" value="It's a bit troublesome"> It's a bit troublesome</label>
</div>
<div class="question">
<h3>8. What is important to you?</h3>
<label><input type="radio" name="q8" value="Family and friends"> Family and friends</label>
<label><input type="radio" name="q8" value="My own time"> My own time</label>
<label><input type="radio" name="q8" value="Money and success"> Money and success</label>
</div>
<div class="question">
<h3>9. How do you spend your day off?</h3>
<label><input type="radio" name="q9" value="Going out and being active"> Going out and being active</label>
<label><input type="radio" name="q9" value="Relaxing at home"> Relaxing at home</label>
<label><input type="radio" name="q9" value="Spending time with friends"> Spending time with friends</label>
</div>
<div class="question">
<h3>10. Are you good at focusing on tasks?</h3>
<label><input type="radio" name="q10" value="I can focus for hours"> I can focus for hours</label>
<label><input type="radio" name="q10" value="Fairly good"> Fairly good</label>
<label><input type="radio" name="q10" value="Long focus is difficult"> Long focus is difficult</label>
</div>
<div id="answer-container" class="answer-container">
<label for="ai-response">AI Response:</label>
<p>*Response may take some time</p>
<textarea id="ai-response" class="ai-response" rows="4" cols="50" readonly></textarea>
</div>
<button type="button" id="button-1731386247">Send to AI</button>
<script>
(() => {
const button = document.getElementById('button-1731386247');
button.addEventListener('click', async () => {
button.disabled = true;
button.style.backgroundColor = '#999';
const selectedOptions = Array.from(document.querySelectorAll('.question')).map((questionDiv) => {
const questionText = questionDiv.querySelector('h3').innerText;
const selectedOption = questionDiv.querySelector('input[type="radio"]:checked');
return selectedOption ? `${questionText}: ${selectedOption.value}` : null;
}).filter(Boolean).join('\n');
if (!selectedOptions) {
alert('Please select one of the options.');
button.disabled = false;
button.style.backgroundColor = '#4CAF50';
return;
}
const serverAi = new ServerAI();
try {
const answer = await serverAi.getAnswerText('uGexdQqzw7DjUEWg7Eq8A2', '', `Questions and selected items:\n${selectedOptions}`);
document.getElementById('ai-response').value = answer;
} catch (error) {
console.error('AI Response Error:', error);
document.getElementById('ai-response').value = 'An error has occurred. Please try again.';
}
button.disabled = false;
button.style.backgroundColor = '#4CAF50';
});
})();
</script>
</body>
</html>